This article shows how to create and call C# msbuild inline task with the UsingTask element. As an example, the UninstallString of the ‘Node.js’ package is searched.
Table of Contents
Create UsingTask Element
Inline Task can use the element ‘UsingTask‘, where C# code with optional Input and Output parameters is specified.
<UsingTask
TaskName="FindUninstallString"
TaskFactory="CodeTaskFactory"
AssemblyFile="$(MSBuildToolsPath)\Microsoft.Build.Tasks.Core.dll" >
<ParameterGroup>
</ParameterGroup>
<Task>
<Reference Include="" />
<Using Namespace="" />
<Code Type="Fragment" Language="cs">
</Code>
</Task>
</UsingTask>
Define Input and Output Parameters
Under UsingTask Element, there is a child element <ParameterGroup> defining Input and Output Parameters.
<ParameterGroup>
<InstalledPackage ParameterType="System.String" Required="true" />
<ReturnString ParameterType="System.String" Output="true" />
</ParameterGroup>
Define C# Code
In this example, the UninstallString for specified Input Parameter ‘InstalledPackage’ will be searched and returned.
<Code Type="Fragment" Language="cs">
<![CDATA[
RegistryKey localMachine = RegistryKey.OpenBaseKey(Microsoft.Win32.RegistryHive.LocalMachine, RegistryView.Registry64);
string regArea = @"SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall";
RegistryKey packagesRoot = localMachine.OpenSubKey(regArea);
string[] packageslisted = packagesRoot.GetSubKeyNames();
string uninstallCommand = String.Empty;
foreach (string p in packageslisted)
{
RegistryKey installProperties = packagesRoot.OpenSubKey(p);
if (installProperties != null)
{
string displayName = (string)installProperties.GetValue("DisplayName");
if ((displayName != null) && displayName.Contains(InstalledPackage))
{
uninstallCommand = (string)installProperties.GetValue("UninstallString");
}
}
}
ReturnString = uninstallCommand ;
]]>
</Code>
Add NameSpace and Reference
Add References and NameSpaces if needed to run the task.
<Using Namespace="Microsoft.Win32"/>
Call the msbuild inline task from Target
The target contains a set of tasks for MSBuild to run, and here this ‘GetUninstallString’ target calls the C# inline Task ‘FindUninstallString’.
<Target Name="GetUninstallString">
<FindUninstallString InstalledPackage="Node.js" >
<Output TaskParameter="ReturnString" ItemName="UninstallStringForPackage"/>
</FindUninstallString>
<Message Text="Unstaill String for Search Product is " />
</Target>
Build the Target
msbuild test.msbuild /t:GetUninstallString
Run:
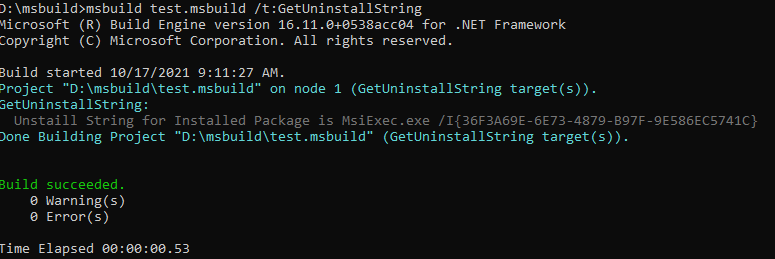
You may be interested in reading more articles: